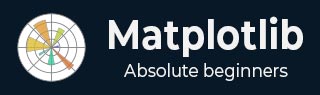
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Working With Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - LaTeX
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Celluloid Library
- Matplotlib - Blitting
- Matplotlib - Toolkits
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Widgets
- Matplotlib - Cursor Widget
- Matplotlib - Annotated Cursor
- Matplotlib - Buttons Widget
- Matplotlib - Check Buttons
- Matplotlib - Lasso Selector
- Matplotlib - Menu Widget
- Matplotlib - Mouse Cursor
- Matplotlib - Multicursor
- Matplotlib - Polygon Selector
- Matplotlib - Radio Buttons
- Matplotlib - RangeSlider
- Matplotlib - Rectangle Selector
- Matplotlib - Ellipse Selector
- Matplotlib - Slider Widget
- Matplotlib - Span Selector
- Matplotlib - Textbox
- Matplotlib Plotting
- Matplotlib - Line Plots
- Matplotlib - Area Plots
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Arrow Demo
- Matplotlib - Fancy Boxes
- Matplotlib - Zorder Demo
- Matplotlib - Hatch Demo
- Matplotlib - Mmh Donuts
- Matplotlib - Ellipse Demo
- Matplotlib - Bezier Curve
- Matplotlib - Bubble Plots
- Matplotlib - Stacked Plots
- Matplotlib - Table Charts
- Matplotlib - Polar Charts
- Matplotlib - Hexagonal bin Plots
- Matplotlib - Violin Plot
- Matplotlib - Event Plot
- Matplotlib - Heatmap
- Matplotlib - Stairs Plots
- Matplotlib - Errorbar
- Matplotlib - Hinton Diagram
- Matplotlib - Contour Plot
- Matplotlib - Wireframe Plots
- Matplotlib - Surface Plots
- Matplotlib - Triangulations
- Matplotlib - Stream plot
- Matplotlib - Ishikawa Diagram
- Matplotlib - 3D Plotting
- Matplotlib - 3D Lines
- Matplotlib - 3D Scatter Plots
- Matplotlib - 3D Contour Plot
- Matplotlib - 3D Bar Plots
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - 3D Vignettes
- Matplotlib - 3D Volumes
- Matplotlib - 3D Voxels
- Matplotlib - Time Plots and Signals
- Matplotlib - Filled Plots
- Matplotlib - Step Plots
- Matplotlib - XKCD Style
- Matplotlib - Quiver Plot
- Matplotlib - Stem Plots
- Matplotlib - Visualizing Vectors
- Matplotlib - Audio Visualization
- Matplotlib - Audio Processing
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Stairs Plots
A stairs plot is a graph used to display data points in a way that highlights changes that occur at distinct intervals or steps. Instead of connecting data points with continuous lines, a stairs plot connects them with horizontal and vertical lines, creating a series of steps.
Imagine you have data points at specific time intervals or discrete events. In a staircase plot, you can draw a horizontal line to represent the value of the data point until the next time interval or event occurs. At that point, you can draw a vertical line to the new value, creating a step in the plot. This process repeats for each data point, resulting in a plot that looks like a staircase −

Stairs Plots in Matplotlib
We can create stair plots in Matplotlib using the step() function. This function connects data points with horizontal and vertical line segments, highlighting the discrete nature of the data.
The step() Function
The step() function in Matplotlib takes parameters for the x and y coordinates of the data points and the linestyle, and is used to represent various types of step plots, such as pre, post, and mid-step.
This function is used to create a step plot, where the values remain constant until the next data point is reached, at which point the value jumps to the new level. It is used to visualize data that changes rapidly at specific points.
Following is the syntax of the step() function in Matplotlib −
matplotlib.pyplot.step(x, y, where='pre', *kwargs)
Where,
- x is the x-coordinates of the data points.
- y is the y-coordinates of the data points.
- where (optional) specifies where the steps should be placed. It can be 'pre' (default), 'post', or 'mid'.
- *kwargs is the additional keyword arguments that is used to customize the appearance of the plot, such as line color, linestyle, and markers.
Basic Stairs Plot
A basic stairs plot in Matplotlib is a visual representation of data that displays changes occurring at specific points, highlighting a stepwise pattern. Each step represents a change in the data, making it easy to visualize transitions over a sequence of values.
Example
In the following example, we are creating a simple stairs plot. We define two lists, 'x' and 'y', as data points. We then use the plt.step() function to generate a step plot using the given data. The resulting graph displays a staircase-like pattern connecting the specified data points on the X and Y axes −
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y = [0, 1, 0, 2, 1] # Creating a basic stairs plot plt.step(x, y, label='Stairs') # Customizing the plot plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Basic Stairs Plot') plt.legend() # Displaying the plot plt.show()
Output
After executing the above code, we get the following output −

Multiple Stairs Plots
A multiple sairs plot in Matplotlib is a visual representation that includes creating and displaying more than one set of stairs plots on the same graph. This allows us to compare multiple datasets or variables within a single plot simultaneously. Each set of stairs represents a distinct dataset, and the combined visualization helps us to highlight patterns, trends, or differences between them.
Example
In here, we are creating a plot with two stair-like patterns. The data includes two sets of Y-values, 'y1' and 'y2', corresponding to the same X-values. The plt.step() function is used to generate two separate stair plots ('Stairs 1' and 'Stairs 2'). The resulting graph displays two distinct staircase patterns along the X and Y axes −
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y1 = [0, 1, 0, 2, 1] y2 = [1, 2, 1, 3, 2] # Creating multiple stairs plots plt.step(x, y1, label='Stairs 1') plt.step(x, y2, label='Stairs 2') # Customizing plot plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Multiple Stairs Plots') plt.legend() # Displaying the plot plt.show()
Output
Following is the output of the above code −

Staircase Plot with Annotation
A staircase plot with annotation in Matplotlib is an extension of the basic stairs plot that includes additional information in the form of annotations. Annotations are text labels placed at specific points along the staircase plot to provide additional details about the data.
Example
Now, we are creating a staircase plot with annotated points. The data defines X and Y values, and the plt.step() function generates the staircase plot. Two specific points on the plot are annotated using plt.text(), indicating a 'Peak' at coordinates (2, 1) and a 'High Point' at coordinates (4, 2) −
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y = [0, 1, 0, 2, 1] # Creating a staircase plot plt.step(x, y, label='Stairs') # Annotate specific points plt.text(2, 1, 'Peak', ha='center', va='bottom', color='red', fontsize=10) plt.text(4, 2, 'High Point', ha='right', va='top', color='blue', fontsize=10) # Customizing plot plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Staircase Plot with Annotation') plt.legend() # Displaying the plot plt.show()
Output
Output of the above code is as follows −

Filled Staircase Plot
A filled staircase plot in Matplotlib is like creating a stairs plot where the area between the steps is filled, creating a visually distinct shape. This type of plot is used to highlight the region between two staircases. To achieve this in matplotlib, we use the fill_between() function.
The "alpha" parameter in the fill_between() function controls the transparency of the filled area, allowing both the stairs plots and the filled region to be visible simultaneously.
Example
In the example below, we are creating two sets of stairs plots "y1" and "y2", and filling the area between them using the fill_between() function −
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y1 = [0, 1, 0, 2, 1] y2 = [0, 2, 1, 3, 2] # Creating a filled staircase plot plt.step(x, y1, label='Stairs 1') plt.step(x, y2, label='Stairs 2') plt.fill_between(x, y1, y2, color='gray', alpha=0.3, label='Filled Area') # Customizing the plot plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Filled Staircase Plot') plt.legend() # Displaying the plot plt.show()
Output
The output obtained is as shown below −
