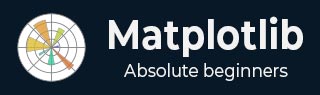
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Working With Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - LaTeX
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Celluloid Library
- Matplotlib - Blitting
- Matplotlib - Toolkits
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Widgets
- Matplotlib - Cursor Widget
- Matplotlib - Annotated Cursor
- Matplotlib - Buttons Widget
- Matplotlib - Check Buttons
- Matplotlib - Lasso Selector
- Matplotlib - Menu Widget
- Matplotlib - Mouse Cursor
- Matplotlib - Multicursor
- Matplotlib - Polygon Selector
- Matplotlib - Radio Buttons
- Matplotlib - RangeSlider
- Matplotlib - Rectangle Selector
- Matplotlib - Ellipse Selector
- Matplotlib - Slider Widget
- Matplotlib - Span Selector
- Matplotlib - Textbox
- Matplotlib Plotting
- Matplotlib - Line Plots
- Matplotlib - Area Plots
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Arrow Demo
- Matplotlib - Fancy Boxes
- Matplotlib - Zorder Demo
- Matplotlib - Hatch Demo
- Matplotlib - Mmh Donuts
- Matplotlib - Ellipse Demo
- Matplotlib - Bezier Curve
- Matplotlib - Bubble Plots
- Matplotlib - Stacked Plots
- Matplotlib - Table Charts
- Matplotlib - Polar Charts
- Matplotlib - Hexagonal bin Plots
- Matplotlib - Violin Plot
- Matplotlib - Event Plot
- Matplotlib - Heatmap
- Matplotlib - Stairs Plots
- Matplotlib - Errorbar
- Matplotlib - Hinton Diagram
- Matplotlib - Contour Plot
- Matplotlib - Wireframe Plots
- Matplotlib - Surface Plots
- Matplotlib - Triangulations
- Matplotlib - Stream plot
- Matplotlib - Ishikawa Diagram
- Matplotlib - 3D Plotting
- Matplotlib - 3D Lines
- Matplotlib - 3D Scatter Plots
- Matplotlib - 3D Contour Plot
- Matplotlib - 3D Bar Plots
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - 3D Vignettes
- Matplotlib - 3D Volumes
- Matplotlib - 3D Voxels
- Matplotlib - Time Plots and Signals
- Matplotlib - Filled Plots
- Matplotlib - Step Plots
- Matplotlib - XKCD Style
- Matplotlib - Quiver Plot
- Matplotlib - Stem Plots
- Matplotlib - Visualizing Vectors
- Matplotlib - Audio Visualization
- Matplotlib - Audio Processing
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Cheatsheet
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Arrow Demo
An "arrow demo" in general is a graphical representation where arrows are used to convey information, point up a direction, or highlight specific points. Arrows are commonly used to indicate vector quantities, movement, or relationships between elements.
For instance, if you are analyzing sales data over several months, you can visually highlight a significant increase in sales during a specific month as shown below −
Arrow Demo in Matplotlib
We can create an arrow demo in Matplotlib using the arrow() function. This function allows you to create arrows on a plot with specified starting and ending points, arrowhead style, color, and linestyle.
The arrow() Function
The arrow() function in Matplotlib takes parameters for the starting point (x, y), the arrow direction (dx, dy), and optional properties such as color and style.
Following is the syntax of the arrow() function in Matplotlib −
matplotlib.pyplot.arrow(x, y, dx, dy, **kwargs)
Where,
- x, y is the starting point of the arrow.
- dx, dy is the change in x and y that defines the arrow direction.
- **kwargs is the additional keyword arguments for customization (e.g., color, linestyle, arrowhead style).
Arrow Demo with Custom Color
We can create an arrow demo with custom color, graphically displaying arrows in a plot, allowing you to choose specific colors to fill the arrowhead.
Example
In the following example, we are creating a arrow starting from the point (0, 0) and pointing in the direction of (1, 1). We then set the arrow to have a red fill color (fc) while keeping a black edge color (ec)−
import matplotlib.pyplot as plt # Starting point x = 0 y = 0 # Arrow direction dx = 1 dy = 1 # Plotting an arrow with a custom color plt.arrow(x, y, dx, dy, head_width=0.05, head_length=0.1, fc='red', ec='black') plt.title('Arrow Demo with Custom Color') plt.show()
Output
After executing the above code, we get the following output −
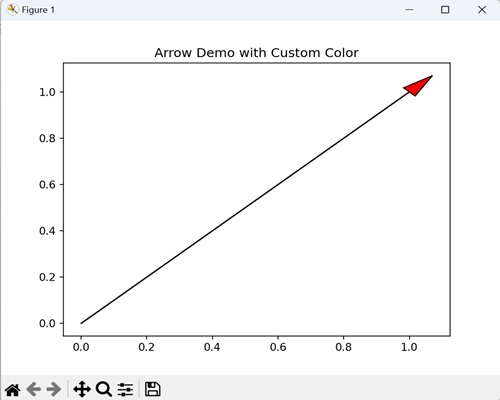
Double-Headed Arrow Demo
A double-headed arrow demo is like drawing a line on a graph, but instead of just one arrowhead at the end, you have arrowheads at both ends. This is useful when you want to show a two-way connection or movement in a plot, making it clear that things go in both directions.
Example
In here, we are creating a double-headed arrow by plotting two arrows: one from (0, 0) to (1, 1) and another in the opposite direction. We are filling the arrows with same fill color (fc) and edge color (ec) −
import matplotlib.pyplot as plt # Starting point x = 0 y = 0 # Arrow direction dx = 1 dy = 1 # Plotting a double-headed arrow plt.arrow(x, y, dx, dy, head_width=0.05, head_length=0.1, fc='green', ec='black') plt.arrow(x+dx, y+dy, -dx, -dy, head_width=0.05, head_length=0.1, fc='green', ec='black') plt.title('Double-Headed Arrow Demo') plt.show()
Output
Following is the output of the above code −
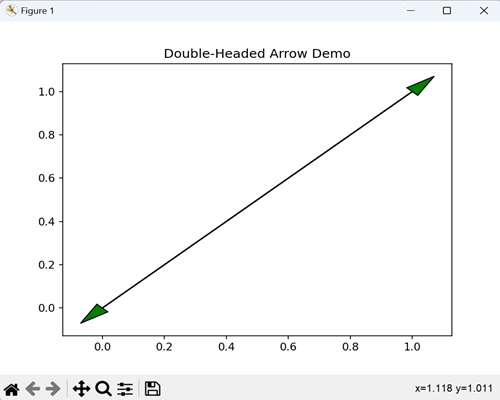
Arrow Demo with Dashed Line
Arrow demo with dashed line refers to outlining the arrow with a dashed line instead of a continuous line. We can draw a dashed line in matplotlib by passing the linestyle argument and setting it to 'dashed' when drawing the arrow.
Example
Now, we are customizing the linestyle of the arrow as a dashed line, having orange fill color and blue edge color −
import matplotlib.pyplot as plt # Starting point x = 0 y = 0 # Arrow direction dx = 1 dy = 1 # Plotting an arrow with a dashed line plt.arrow(x, y, dx, dy, head_width=0.05, head_length=0.1, fc='orange', ec='blue', linestyle='dashed') plt.title('Arrow Demo with Dashed Line') plt.show()
Output
Output of the above code is as follows −
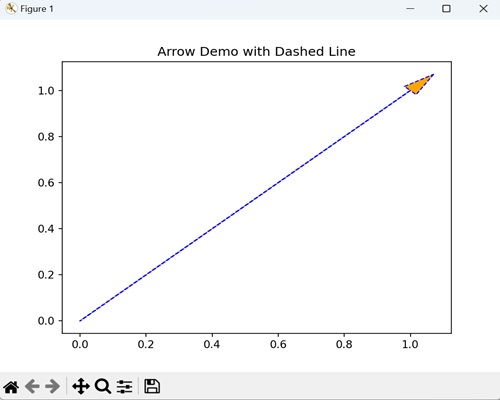
Arrow Demo with Custom Arrowhead Shape
While creating an arrow in a plot, we can also customize the shape of the arrowheads. Instead of the standard full arrowhead, you can choose a unique shape, like a right-pointing triangle or a left-pointing triangle, positioned at the right-half or the left-half respectively at the end of the arrow line.
Example
In the example below, we are customizing the arrowhead shape to be a right-pointing triangle i.e. the arrowhead is positioned at the right end of the arrow line −
import matplotlib.pyplot as plt # Starting point x = 0 y = 0 # Arrow direction dx = 1 dy = 1 # Plotting an arrow with a custom arrowhead shape plt.arrow(x, y, dx, dy, head_width=0.05, head_length=0.1, fc='purple', ec='green', shape='right') plt.title('Arrow Demo with Custom Arrowhead Shape') plt.show()
Output
The output obtained is as shown below −
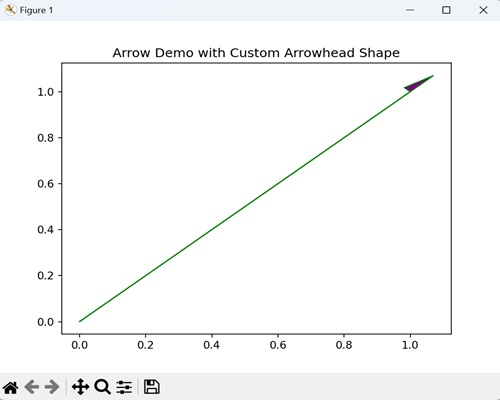