Full-stack React framework for Spring
Benefits of pairing React and Spring
Boot with Hilla
File-based routing
src/main/frontend/views
folder. Hilla will automatically register the route and capture parameters. You can also configure properties like view title and access control right in your React component.
// views/products/{id}/edit.tsx
export const config: ViewConfig = {
title: "Edit Product",
rolesAllowed: ["ADMIN"]
}
export default function ProductEditor() {
const {id} = useParams();
return (
<div>
<h2>Edit product {id}</h2>
...
</div>
);
};
Call Java services directly from React
Hilla enables developers to use React with Java. Use @BrowserCallable
to make a Spring class available to your browser. Hilla uses Spring Security to ensure that only the right people have access.
// Spring Boot
@BrowserCallable
class CustomerService {
private final CustomerRepo repo;
CustomerService(CustomerRepo repo) {
this.repo = repo;
}
public List<Customer> getCustomers() {
repo.findAll();
}
}
// React
function CustomerList() {
// Customer type is automatically generated by Hilla
const customers = useSignal<Customer[]>([]);
useEffect(() => {
CustomerService.getCustomers().then(newCustomers =>
customers.value = newCustomers
);
}, []);
return (
<ComboBox items={customers.value} />
)
}
Move fast without breaking stuff with end-to-end type safety
Spend less time reading API docs and more time coding. Automatically generated TypeScript services and data types allow you to explore APIs right in your IDE.
Breaking changes to the API leads to compile-time errors instead of runtime errors in the frontend.
.png)
Ensure data consistency with shared form validation logic
Define validation rules in the Java model object, Hilla will automatically reuse them in your React form. On form submit, Hilla automatically re-validates the input in your Spring service.
public class Customer {
@NotBlank(message = "Name is mandatory")
private String name;
@NotBlank(message = "Email is mandatory")
@Email
private String email;
// Getters and setters
}
The Hilla useForm hook uses the generated TypeScript model to apply the validation rules to the form fields.
function CustomerForm() {
const {model, field, submit} = useForm(CustomerModel, {
onSubmit: CustomerService.saveCustomer
});
return (
<div>
<TextField label="Name" {...field(model.name)} />
<EmailField label="Email" {...field(model.email)} />
<Button onClick={submit}>Save</Button>
</div>
)
}
UI components included
Hilla uses the Vaadin UI component library designed for complex, data-heavy business applications. Easily customize forms, data grids, editors, and more to fit your brand. All components are rigorously tested to ensure WCAG 2.2 AA accessibility.
No lock-in: Hilla supports any React components, so you can also bring your own or mix and match as needed.
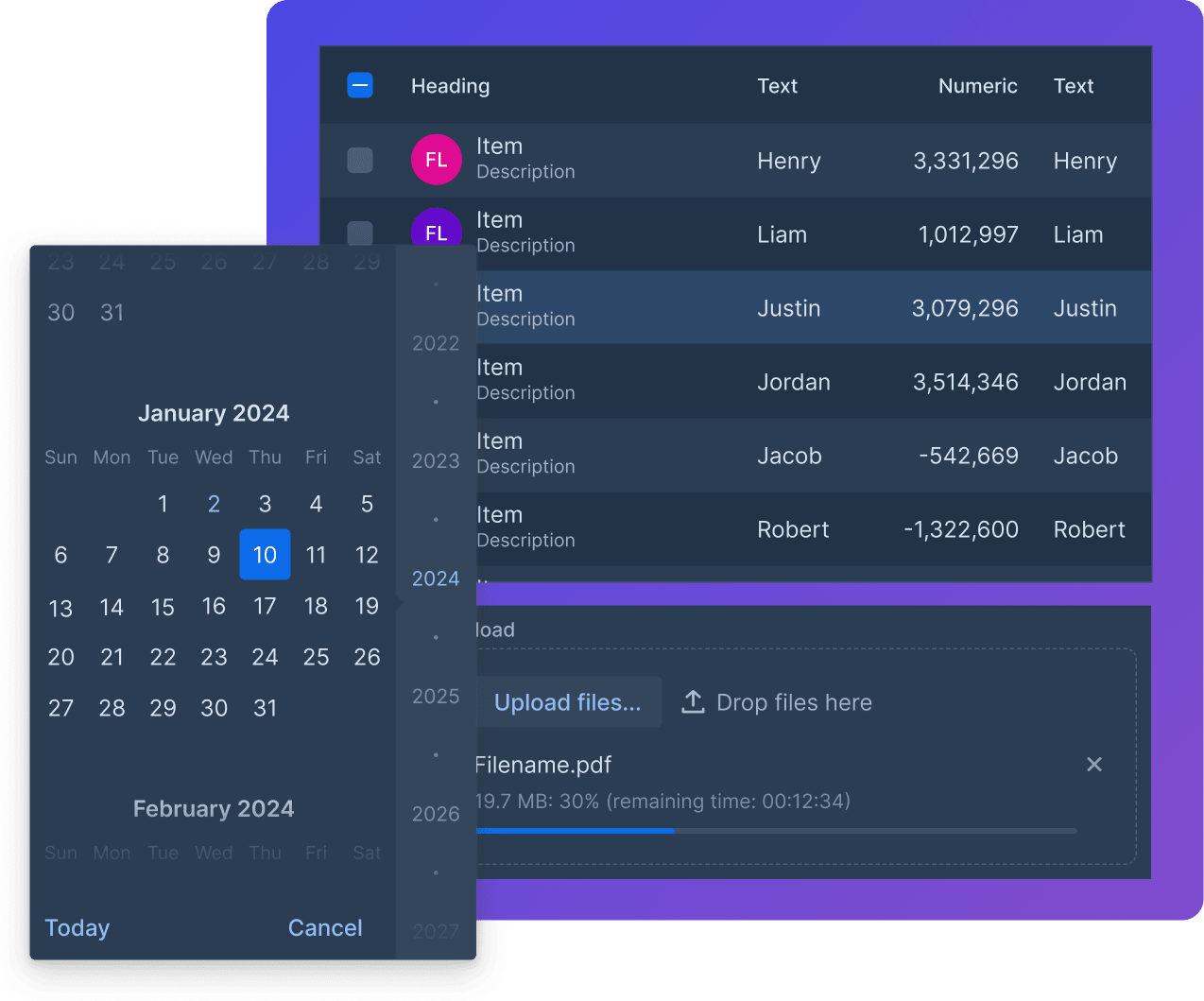
Stream live updates to the browser with ease
With support for reactive data types, you can build real-time dashboards, notifications, and more without managing websockets yourself.
To stream data from the Spring Boot backend to the React frontend, return a Project Reactor Flux data type from your service.
@BrowserCallable
@Service
public class CustomerService {
public Flux<Customer> getOnlineCustomers() {
return Flux.just(/*...Online customers...*/);
}
}
Then subscribe to the stream and update the client state as new data comes in.
function OnlineCustomers() {
const [onlineCustomers, setOnlineCustomers] = useState<Customer[]>([]);
// Instead of awaiting a single response, subscribe to the stream of data
useEffect(() => {
CustomerService.getOnlineCustomers().onNext(setOnlineCustomers);
}, []);
return (
<AvatarGroup
items={onlineCustomers.map((customer) => ({
name: customer.name,
img: customer.profilePicture
}))} />
)
}
What are developers saying?
a go-to solution for combining React and Spring Boot and building robust business applications.
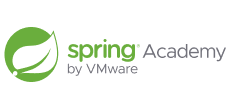
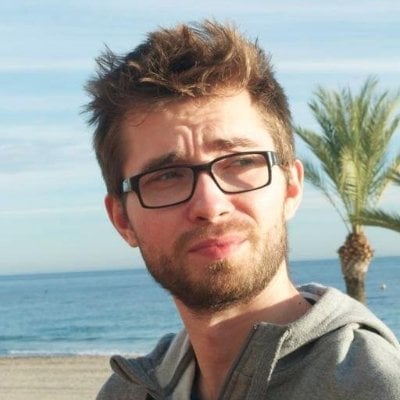
Maciej Walkowiak
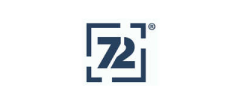
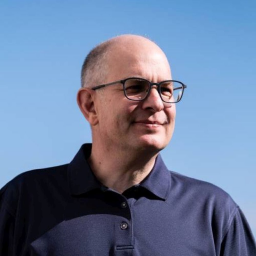
Simon Martinelli
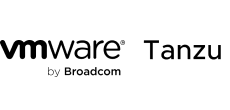
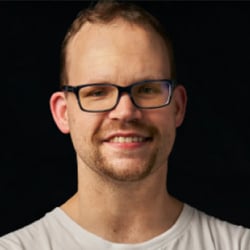
Josh Long
More reasons to choose Hilla
Powerful forms with shared validations
Stateless and scalable
Secure communication out-of-the-box
Built-in support for streaming data
Works with React and Lit frontends
TypeScript autocompletion
Truly open source
Designed for Spring Boot
Enterprise support options
Your brand, your look
Accessible components
Single Sign-On Support
GraalVM native image support
Installable on any device
Ready to build better business applications, faster?
Get up and running in minutes.