Nx plugin to use Go in a Nx workspace.
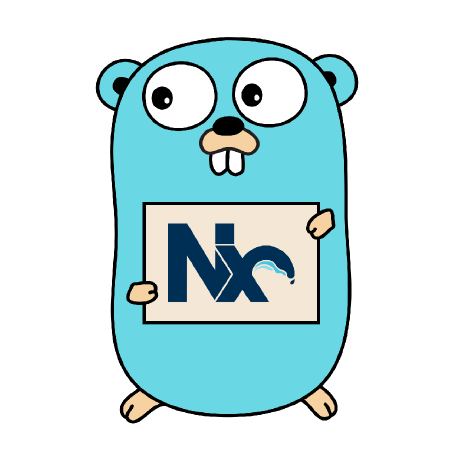
Using nx-go in your company? Consider sponsoring me and get priority support in the issues.
First, make sure you have a Nx Workspace.
Create a new one using the following command:
pnpm dlx create-nx-workspace go-playground --preset=empty --cli=nx --nx-cloud true
## Or using yarn
# yarn create nx-workspace go-playground --preset=empty --cli=nx --nx-cloud true
## Or using npm
# npm exec create-nx-workspace go-playground --preset=empty --cli=nx --nx-cloud true
cd go-playground
Next, install the nx-go plugin:
pnpm add -D @nx-go/nx-go
## Or using yarn
# yarn add -D @nx-go/nx-go
## Or using npm
# npm install -D @nx-go/nx-go
Create a new application:
nx g @nx-go/nx-go:app api
nx-go includes a plugin that will build the dependency graph of go modules within your nx workspace. This is not enabled by default and must be enabled explicitly.
To enable the nx-go plugin for the dependency graph run the following command:
nx generate @nx-go/nx-go:setup-nx-go-plugin
You can now run the Nx workspace commands:
This command builds the application using the go build
command, and stores the output in the dist/<app-name>/
directory.
nx build api
Lint the application using the go fmt
command.
nx lint api
You can define a custom linter that will execute instead of the default go fmt
.
The lint executor provides two options to configure the desired linter:
interface LintOptions {
linter: string
args: string
}
linter: is the command to execute (example: revive
)
args: these are additional arguments to be supplied to the linter (example: -config revive.toml
)
The examples above will result in the following command that will be executed:
revive -config revive.toml ./...
Serves the application using the go run
command.
nx serve api
To run the application in watch mode you can use gow
, after installing it on your machine.
Open the file apps/<app-name>/project.json
and in the targets.serve.options
object and set the cmd
parameter to gow
and the cwd
parameter to .
, like so:
{
targets: {
serve: {
executor: '@nx-go/nx-go:serve',
options: {
cmd: 'gow', // This is the cmd that will be used
cwd: '.', // Set working dir to project root so it picks up changes in `libs/*`
main: 'apps/api/main.go',
},
},
},
}
Test the application using the go test
command.
nx test api
In order to build Docker containers from the Go api inside the Nx Workspace, there are 2 base images provided:
- nxgo/base
- Node 14 on Alpine, with Go 1.13
- nxgo/cli
- Node 14 on Alpine, with Go 1.13
- @angular/cli v10
- @nrwl/cli v10
- nxpm v1
# Use nxgo/cli as the base image to do the build
FROM nxgo/cli as builder
# Create app directory
WORKDIR /workspace
# Copy package.json and the lock file
COPY package.json yarn.lock /workspace/
# Install app dependencies
RUN yarn
# Copy source files
COPY . .
# Build apps
RUN yarn build api
# This is the stage where the final production image is built
FROM golang:1.17-alpine as final
# Copy over artifacts from builder image
COPY --from=builder /workspace/dist/apps/api /workspace/api
# Set environment variables
ENV PORT=3000
ENV HOST=0.0.0.0
# Expose default port
EXPOSE 3000
# Start server
CMD [ "/workspace/api" ]
Created by Bram Borggreve.