Accept a payment
Securely accept payments online.
Build a payment form or use a prebuilt checkout page to start accepting online payments.
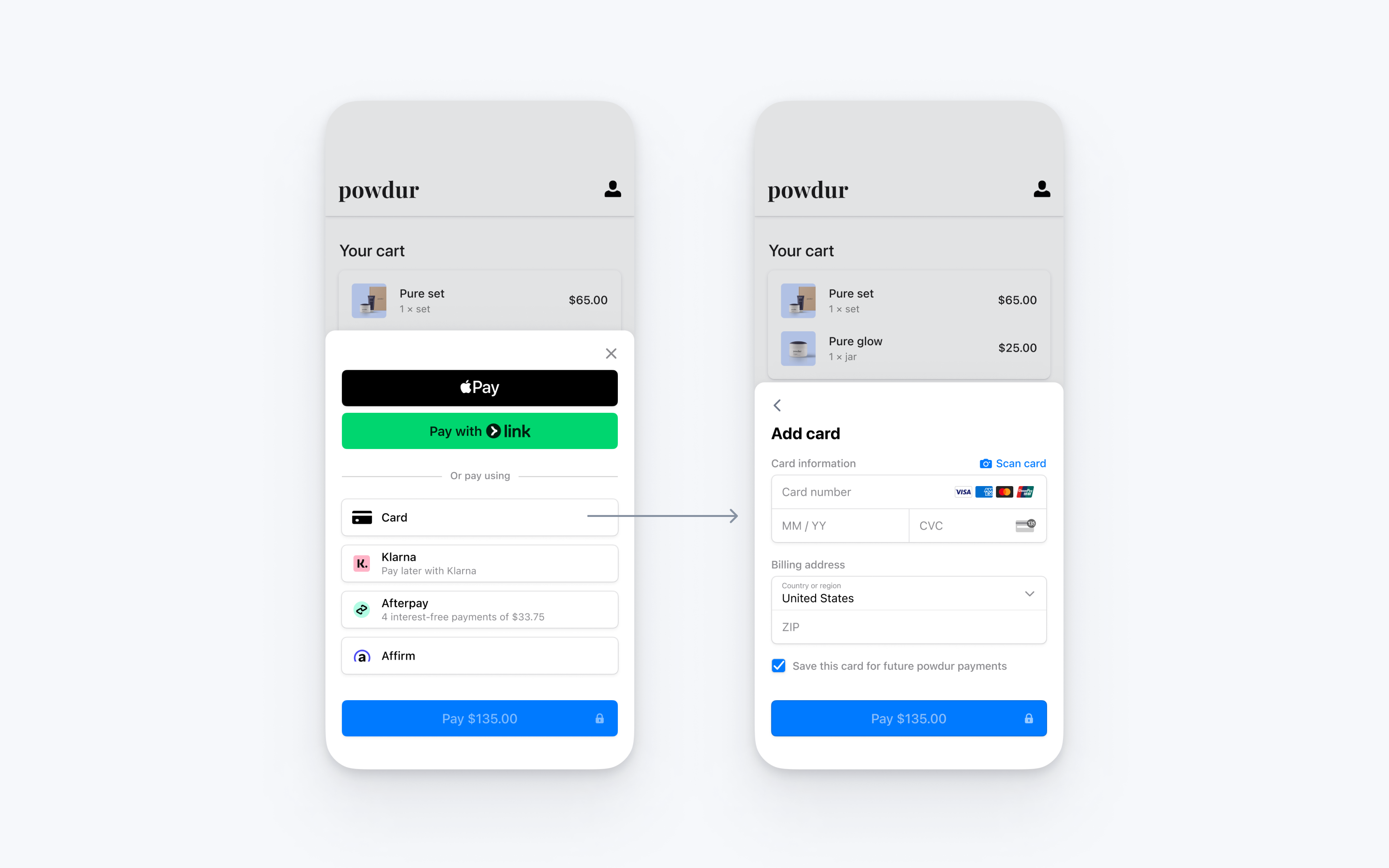
Integrate Stripe’s prebuilt payment UI into the checkout of your iOS app with the PaymentSheet class. See our sample integration on GitHub.
Interested in displaying payment methods directly in your checkout screen?
We’re exploring an integration that lets you embed a prebuilt view directly in your checkout to display payment methods. Sign up for early access.
Set up StripeServer-sideClient-side
First, you need a Stripe account. Register now.
Server-side 
This integration requires endpoints on your server that talk to the Stripe API. Use our official libraries for access to the Stripe API from your server:
Client-side 
The Stripe iOS SDK is open source, fully documented, and compatible with apps supporting iOS 13 or above.
Note
For details on the latest SDK release and past versions, see the Releases page on GitHub. To receive notifications when a new release is published, watch releases for the repository.
Enable payment methods
View your payment methods settings and enable the payment methods you want to support. You need at least one payment method enabled to create a PaymentIntent.
By default, Stripe enables cards and other prevalent payment methods that can help you reach more customers, but we recommend turning on additional payment methods that are relevant for your business and customers. See Payment method support for product and payment method support, and our pricing page for fees.
Add an endpointServer-side
Note
If you want to present the PaymentSheet before creating a PaymentIntent, see Collect payment details before creating an Intent.
This integration uses three Stripe API objects:
PaymentIntent: Stripe uses this to represent your intent to collect payment from a customer, tracking your charge attempts and payment state changes throughout the process.
(Optional) Customer: To set up a payment method for future payments, you must attach it to a Customer. Create a Customer object when your customer creates an account with your business. If your customer is making a payment as a guest, you can create a Customer object before payment and associate it with your own internal representation of the customer’s account later.
(Optional) Customer Ephemeral Key: Information on the Customer object is sensitive, and can’t be retrieved directly from an app. An Ephemeral Key grants the SDK temporary access to the Customer.
Note
If you never save cards to a Customer and don’t allow returning Customers to reuse saved cards, you can omit the Customer and Customer Ephemeral Key objects from your integration.
For security reasons, your app can’t create these objects. Instead, add an endpoint on your server that:
- Retrieves the Customer, or creates a new one.
- Creates an Ephemeral Key for the Customer.
- Creates a PaymentIntent with the amount, currency, and customer. You can also optionally include the
automatic_
parameter. Stripe enables its functionality by default in the latest version of the API.payment_ methods - Returns the Payment Intent’s client secret, the Ephemeral Key’s
secret
, the Customer’s id, and your publishable key to your app.
The payment methods shown to customers during the checkout process are also included on the PaymentIntent. You can let Stripe pull payment methods from your Dashboard settings or you can list them manually. Regardless of the option you choose, know that the currency passed in the PaymentIntent filters the payment methods shown to the customer. For example, if you pass eur
on the PaymentIntent and have OXXO enabled in the Dashboard, OXXO won’t be shown to the customer because OXXO doesn’t support eur
payments.
Unless your integration requires a code-based option for offering payment methods, Stripe recommends the automated option. This is because Stripe evaluates the currency, payment method restrictions, and other parameters to determine the list of supported payment methods. Payment methods that increase conversion and that are most relevant to the currency and customer’s location are prioritized.
Collect payment detailsClient-side
To display the mobile Payment Element on your checkout screen, make sure you:
- Display the products the customer is purchasing along with the total amount
- Use the Address Element to collect any required shipping information from the customer
- Add a checkout button to display Stripe’s UI
If PaymentSheetResult
is .
, inform the user (for example, by displaying an order confirmation screen).
Setting allowsDelayedPaymentMethods
to true allows delayed notification payment methods like US bank accounts. For these payment methods, the final payment status isn’t known when the PaymentSheet
completes, and instead succeeds or fails later. If you support these types of payment methods, inform the customer their order is confirmed and only fulfill their order (for example, ship their product) when the payment is successful.
Set up a return URLClient-side
The customer might navigate away from your app to authenticate (for example, in Safari or their banking app). To allow them to automatically return to your app after authenticating, configure a custom URL scheme and set up your app delegate to forward the URL to the SDK. Stripe doesn’t support universal links.
Additionally, set the returnURL on your PaymentSheet.Configuration object to the URL for your app.
var configuration = PaymentSheet.Configuration() configuration.returnURL = "your-app://stripe-redirect"
Handle post-payment eventsServer-side
Stripe sends a payment_intent.succeeded event when the payment completes. Use the Dashboard webhook tool or follow the webhook guide to receive these events and run actions, such as sending an order confirmation email to your customer, logging the sale in a database, or starting a shipping workflow.
Listen for these events rather than waiting on a callback from the client. On the client, the customer could close the browser window or quit the app before the callback executes, and malicious clients could manipulate the response. Setting up your integration to listen for asynchronous events is what enables you to accept different types of payment methods with a single integration.
In addition to handling the payment_
event, we recommend handling these other events when collecting payments with the Payment Element:
Event | Description | Action |
---|---|---|
payment_intent.succeeded | Sent when a customer successfully completes a payment. | Send the customer an order confirmation and fulfill their order. |
payment_intent.processing | Sent when a customer successfully initiates a payment, but the payment has yet to complete. This event is most commonly sent when the customer initiates a bank debit. It’s followed by either a payment_ or payment_ event in the future. | Send the customer an order confirmation that indicates their payment is pending. For digital goods, you might want to fulfill the order before waiting for payment to complete. |
payment_intent.payment_failed | Sent when a customer attempts a payment, but the payment fails. | If a payment transitions from processing to payment_ , offer the customer another attempt to pay. |
Test the integration
See Testing for additional information to test your integration.